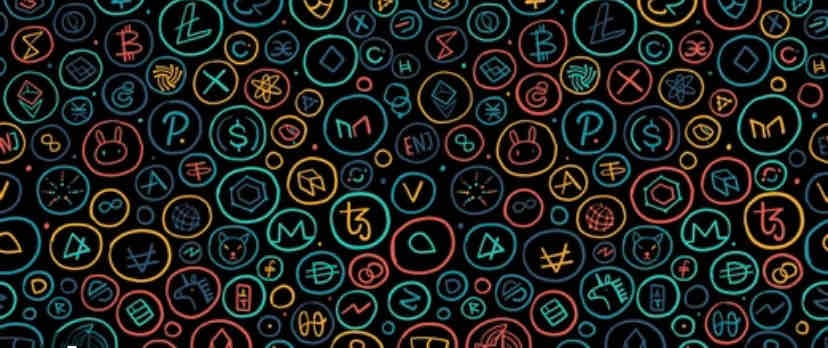
Glib
@sushev
108 Following
12 Followers
0 reply
0 recast
0 reaction
0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction
Welcome Speech for My Blog
Hello, dear readers!
Welcome to my blog, a space where ideas spark, stories unfold, and inspiration takes root. Whether you're here to explore new perspectives, dive into practical tips, or simply enjoy a good read, Iโm thrilled to have you along for the journey. This blog is all about sharing knowledge, sparking curiosity, and building a community of like-minded souls. So, grab a cozy spot, letโs dive into the adventure together, and make every moment here count!
Stay tuned for more,
๐ ๐ ๐ ๐ฅฐ ๐ ๐คฃ 0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction
0 reply
0 recast
0 reaction
0 reply
0 recast
0 reaction
0 reply
0 recast
0 reaction
0 reply
0 recast
0 reaction
0 reply
0 recast
0 reaction
0 reply
0 recast
0 reaction
Define Objectives
Trading Goals:
Decide what the bot should achieve (e.g., scalping, trend following, arbitrage).
Market and Assets:
Choose the market (e.g., stocks, cryptocurrency, forex) and the assets your bot will trade.
Risk Management:
Set rules for position sizing, stop-loss limits, and risk tolerance.
2. Understand the Basics
Market Knowledge: Learn about market structures, trading strategies, and indicators.
Programming Skills: Proficiency in a programming language like Python, JavaScript, or C++ is essential.
APIs: Familiarize yourself with APIs of trading platforms (e.g., Binance, Coinbase, Interactive Brokers).
Regulations: Ensure compliance with trading regulations in your jurisdiction. 0 reply
0 recast
0 reaction
1. Junior Developer (Entry-Level)
Experience: 0โ2 years
Key Characteristics:
Learning the basics of coding, tools, and processes.
Requires guidance and supervision from senior team members.
Works on well-defined tasks, such as fixing bugs or implementing small features.
Focuses on learning company-specific tech stacks, best practices, and workflows.
Skills:
Basic knowledge of programming languages and frameworks.
Familiarity with version control systems (e.g., Git).
Eager to learn and improve.
2. Mid-Level Developer (Intermediate)
Experience: 2โ5 years
Key Characteristics:
Capable of handling tasks independently with minimal supervision.
Works on more complex features, integrations, and improvements.
Begins to mentor junior developers.
Balances speed and quality in code delivery.
Skills:
Proficient in one or more programming languages or frameworks.
Good understanding of software development life cycles.
Knowledge of debugging, testing, and optimization. 0 reply
0 recast
1 reaction
Certainly! Let's explore Mocha, a popular JavaScript test framework.
Mocha
What it is: Mocha is a feature-rich JavaScript test framework that runs on Node.js and in the browser. It provides a flexible and expressive syntax for writing tests.
Key Features:
Supports various testing styles: Mocha supports different testing styles, including:
BDD (Behavior-Driven Development): Using describe and it blocks to define tests in a human-readable format.
Reporting: Provides various reporting options, including console output, JUnit XML, and HTML reports.
Parallel Testing: Can run tests in parallel for faster execution.
Example:
JavaScript
const assert = require('assert');
describe('Math Operations', function() {
it('should add two numbers correctly', function() {
assert.equal(2 + 2, 4);
});
it('should subtract two numbers correctly', function() {
assert.equal(5 - 3, 2);
});
}); 0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction
0 reply
0 recast
1 reaction